Setting Up Your Development Environment
Creating a basic HTML5 landing page requires not just knowledge of HTML but also an understanding of how to set up your development environment effectively. This foundational step ensures that you have the right tools and resources necessary to begin coding efficiently.
Firstly, it is essential to choose a text editor or Integrated Development Environment (IDE) that suits your workflow. Text editors like Visual Studio Code, Sublime Text, and Atom are popular choices among developers for their simplicity and powerful features. These editors offer extensions such as live server previews, syntax highlighting, code snippets, and linting tools which streamline the development process. IDEs like WebStorm or Eclipse provide more advanced features, including project management capabilities and debugging tools, making them ideal for larger projects.
Secondly, familiarizing yourself with basic HTML5 structure is crucial. Every HTML document begins with a doctype declaration followed by the <html>
tag which acts as the root element of an HTML page. Inside this, you will have two main sections: <head>
and <body>
. The <head>
section contains metadata about your webpage such as its title, character set definition, CSS links, scripts, and more. This part ensures that browsers understand how to render content correctly. The <body>
section is where all visible elements of the page are placed.
Lastly, setting up version control with Git is a best practice for any developer working on web projects. Version control allows you to track changes in your files over time, facilitating collaboration and enabling easy rollback in case something goes wrong during development. GitHub provides free hosting services alongside an intuitive interface for managing repositories. By initializing a new repository locally using git init
, committing changes with descriptive messages via git commit -m "message"
, and pushing these commits to GitHub using git push
, developers ensure their projects remain organized and accessible.
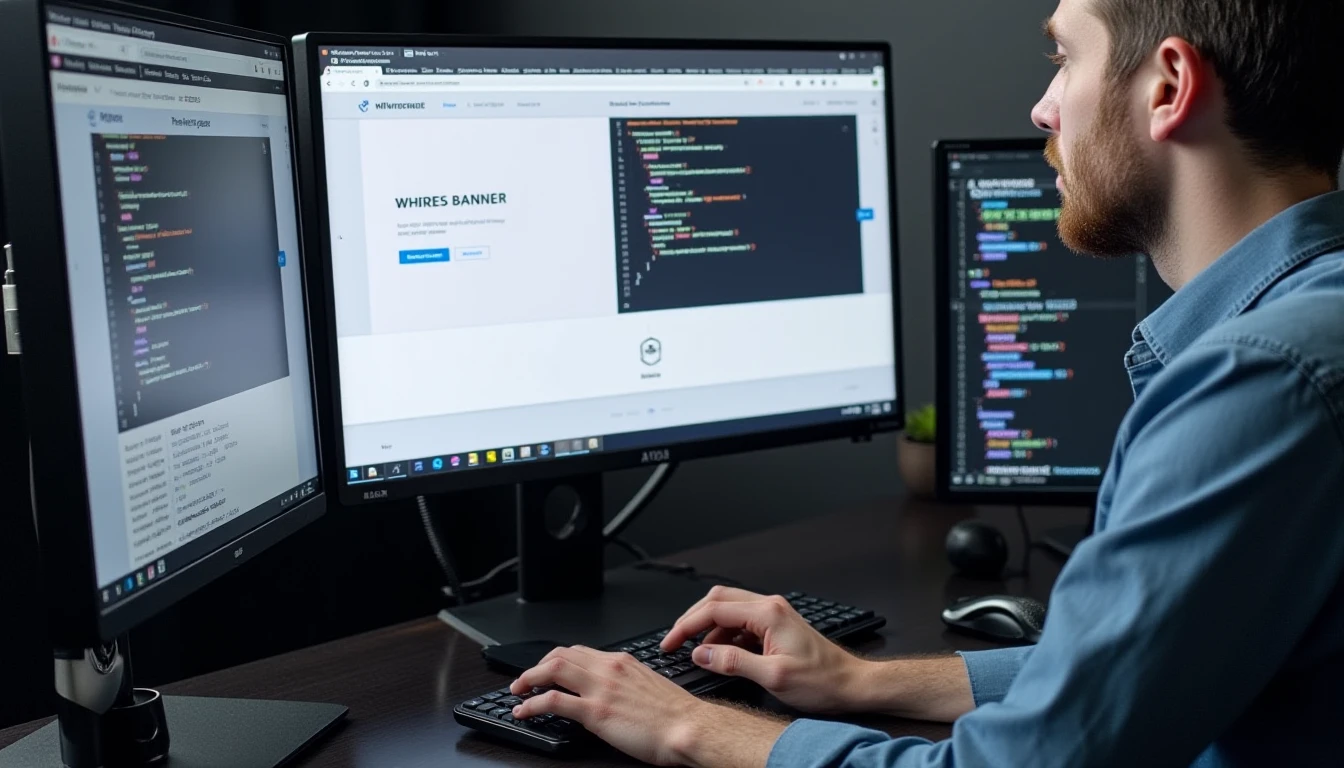
Designing the Layout
Designing the layout of your HTML5 landing page involves structuring content in a way that is both visually appealing and functional. This part focuses on defining how elements should be arranged on screen, considering factors like responsiveness and accessibility to create an engaging user experience.
To start with, understanding CSS Grid and Flexbox is vital for designing layouts efficiently. Both these systems offer flexible ways to define container areas where child elements can be placed. While Flexbox excels at one-dimensional alignment (either rows or columns), CSS Grid provides more control over two-dimensional layout designs, making it suitable for complex structures like those found in landing pages. Utilizing media queries within your stylesheet allows you to adapt these layouts based on different screen sizes and orientations, ensuring optimal viewing experiences across various devices.
Moreover, incorporating semantic HTML tags enhances accessibility by providing meaningful context about the structure of the document. Tags such as <header>
, <nav>
, <main>
, <article>
, <section>
, <aside>
, and <footer>
help search engines understand what each section represents, aiding in better SEO ranking while also improving navigation for screen readers used by visually impaired users. Proper use of heading tags (e.g., <h1>
, <h2>
) ensures logical document flow which is crucial for accessibility compliance.
Lastly, integrating a responsive design framework can significantly simplify the process of creating mobile-friendly layouts. Frameworks like Bootstrap offer pre-designed components and utility classes that make it easier to implement consistent styling across all screens without needing extensive custom CSS code. These frameworks often come with grid systems, navigation bars, modals, buttons, etc., ready for immediate use, reducing development time while maintaining a high standard of design quality.
Adding Interactive Elements
Adding interactive elements to your HTML5 landing page elevates its functionality and user engagement levels. This section explores various methods to incorporate interactivity using JavaScript, ensuring that users have more than just static content to interact with.
JavaScript forms the core foundation for adding dynamic behavior to web pages. With libraries like jQuery or vanilla JS (plain JavaScript), developers can manipulate DOM elements in real-time. For instance, toggling visibility of hidden sections, handling form submissions via AJAX requests, or dynamically updating page content based on user input are common applications that enhance usability and reduce server load.
Implementing event listeners is key to creating responsive interactions without reloading pages entirely. By attaching functions to specific events (such as clicks, hovers, or focus changes), developers can trigger actions seamlessly when users perform certain tasks. For example, adding an “Open Menu” button on a mobile layout that toggles between showing and hiding navigation links exemplifies this concept effectively. Libraries like jQuery simplify event handling by providing concise syntax for binding events to elements, making it easier for less experienced coders to implement robust interactivity.
Lastly, leveraging modern web APIs can introduce cutting-edge features into your landing page. The Fetch API allows asynchronous HTTP requests to communicate with servers without blocking the main thread, enabling seamless data fetching operations that update UI components instantly. Similarly, Web Storage API provides mechanisms to store key-value pairs locally within browsers (session storage for current session or local storage across sessions), useful for remembering user preferences or maintaining state information between page reloads.
Enhancing Visual Appeal with CSS
Enhancing the visual appeal of your HTML5 landing page through CSS involves more than just applying colors and fonts; it encompasses creating a harmonious design that aligns with brand identity while ensuring readability and accessibility. This section delves into essential aspects of styling web pages to achieve professional results.
Choosing appropriate typography is fundamental for setting the right tone on any webpage. Web-safe fonts ensure broad browser compatibility, but leveraging tools like Google Fonts expands options significantly. Selecting a primary font family that complements your brand’s personality while providing sufficient contrast against background colors ensures text remains legible across devices. Pairing complementary secondary fonts can add visual interest without overwhelming readability.
Responsive design techniques are crucial for adapting layouts to diverse screen sizes gracefully. Media queries enable conditional styling based on breakpoints defined by viewport width, enabling adjustments in layout structures, padding margins, and font sizes as needed. Utilizing relative units like percentages or ems instead of absolute values ensures that elements scale appropriately regardless of device resolution. Additionally, flexible grid systems utilizing CSS Grid or Flexbox allow for dynamic resizing of content areas maintaining balance even on narrow screens.
Accessibility considerations should never be overlooked when styling web pages. Ensuring sufficient color contrast between text and background colors improves visibility for visually impaired users. Applying appropriate heading structures helps screen readers navigate documents logically. Implementing ARIA roles where necessary provides additional context about interactive elements like buttons, sliders, or tabs aiding assistive technologies in guiding users through the interface effectively.
Optimizing Performance
Optimizing performance is critical for ensuring your HTML5 landing page loads quickly and runs smoothly across devices, contributing positively to user experience and SEO rankings. This final section examines strategies for improving load times and enhancing overall efficiency.
Minifying CSS and JavaScript files removes unnecessary characters such as whitespace, comments, and line breaks without affecting functionality, thereby reducing file sizes and download times. Tools like UglifyJS for JS or CleanCSS for CSS automate this process efficiently, saving manual effort while delivering significant performance gains. Additionally, combining multiple CSS/JS files into one reduces HTTP requests which can otherwise slow down page loading speeds.
Image optimization plays a pivotal role in enhancing site speed as images often contribute significantly to total file size. Using modern formats like WebP alongside lossy compression techniques ensures high-quality visuals without excessive bloat. Implementing lazy loading delays image rendering until they are within the viewport, further decreasing initial load times by prioritizing essential content first. Tools such as ImageOptim or TinyPNG offer easy-to-use interfaces for batch processing images ensuring quick turnaround while maintaining quality standards.
Finally, leveraging browser caching allows frequently accessed resources to be stored locally temporarily improving subsequent visits’ performance. By specifying cache-control headers in your server configuration, you instruct browsers on how long assets should remain cached before checking for updates. This approach not only speeds up repeat access but also reduces bandwidth usage beneficial both environmentally and financially over time.